Query data from the IIASA database infrastructure¶
The IIASA Energy, Climate, and Environment Program hosts a suite of Scenario Explorer instances and related database infrastructure to support analysis of integrated-assessment pathways in IPCC reports and model comparison projects. High-profile use cases include the AR6 Scenario Explorer hosted by IIASA supporting the IPCC’ Sixth Assessment Report (AR6) and the Horizon 2020 project ENGAGE.
IIASA’s modeling platform infrastructure and the scenario apps are not only a great resource on its own, but it also allows the underlying datasets to be queried directly via a Rest API. The pyam package takes advantage of this ability to allow you to easily pull data and work with it in your Python data processing and analysis workflow.
Access and permission management for project-internal databases¶
By default, your can connect to all public scenario database instances.
ixmp4 login <username>
You will be prompted to enter your password.
Your username and password will be saved locally in plain-text for future use!
When connecting to a database, pyam will automatically search for the configuration in a known location.
[1]:
import pyam
Connecting to ixmp4 database instances hosted by IIASA¶
The Scenario Apps use the ixmp4 package as a database backend.
You can list all available ixmp4 platforms hosted by IIASA using the following function.
[2]:
pyam.iiasa.platforms()
[INFO] 13:05:26 - ixmp4.conf.auth: Connecting to service anonymously and without credentials.
Platforms accessible via 'https://api.manager.ece.iiasa.ac.at/v1'
Name Access Notice
public-test
public
This is a public ixmp4 test instance hosted by the IIA...
ecemf
public
genie
public
This is a development instance for the GENIE knowledge...
ssp-extensions
public
ssp
public
This platform has the "basic drivers", i.e., GDP and p...
Total: 5
Connecting to a (legacy) Scenario Explorer database¶
Accessing the database connected to a Scenario Explorer is done via a Connection object.
[3]:
conn = pyam.iiasa.Connection()
conn.valid_connections
[INFO] 13:05:27 - pyam.iiasa: You are connected as an anonymous user
[WARNING] 13:05:27 - pyam.iiasa: IIASA is migrating to a database infrastructure using the ixmp4 package.Use `pyam.iiasa.platforms()` to list available ixmp4 databases.
[3]:
['ngfs_phase_3',
'iamc15',
'integration-test',
'cdlinks',
'india_scenario_hub',
'genie',
'hotspots',
'commit',
'kopernikus_public',
'nexus-basins',
'ngfs_phase_4',
'senses',
'aqnea',
'navigate',
'climate_solutions',
'eu-climate-advisory-board',
'engage',
'nca5',
'openentrance',
'ar6-public',
'deeds',
'ngfs_phase_2',
'gei',
'netzero2040',
'ripples',
'ssp',
'ecemf',
'set_nav',
'cmin',
'ariadne',
'paris_lttg']
Reading data from a database hosted by IIASA¶
In this example, we will be retrieving data from the IAMC 1.5°C Scenario Explorer hosted by IIASA (link), which provides the quantitative scenario ensemble underpinning the IPCC Special Report on Global Warming of 1.5C (SR15).
This can be done either via the constructor:
pyam.iiasa.Connection('iamc15')
or, if you want to query multiple databases, via the explicit connect()
method:
conn = pyam.iiasa.Connection()
conn.connect('iamc15')
We also provide some convenience functions to shorten the amount of code you have to write. Under the hood, read_iiasa()
is just opening a connection to a database API and sends a query to the resource.
In this tutorial, we will query specific subsets of data in a manner similar to pyam.IamDataFrame.filter()
.
[4]:
df = pyam.read_iiasa(
"iamc15",
model="MESSAGEix*",
variable=["Emissions|CO2", "Primary Energy|Coal"],
region="World",
meta=["category"],
)
[INFO] 13:05:28 - pyam.iiasa: You are connected to the IXSE_SR15 scenario explorer hosted by IIASA. If you use this data in any published format, please cite the data as provided in the explorer guidelines: https://data.ece.iiasa.ac.at/iamc-1.5c-explorer/#/about
[INFO] 13:05:28 - pyam.iiasa: You are connected as an anonymous user
Here we pulled out all times series data for model(s) that start with ‘MESSAGEix’ that are in the ‘World’ region and associated with the two named variables. We also added the meta column “category”, which tells us the climate impact categorisation of each scenario as assessed in the IPCC SR15.
Let’s plot CO2 emissions.
[5]:
ax = df.filter(variable="Emissions|CO2").plot(
color="category", legend=dict(loc="center left", bbox_to_anchor=(1.0, 0.5))
)
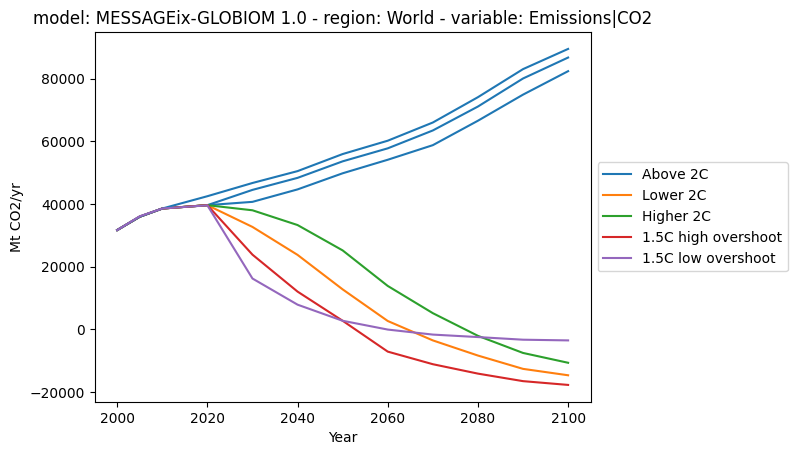
And now continue doing all of your analysis!
[6]:
ax = df.plot.scatter(
x="Primary Energy|Coal",
y="Emissions|CO2",
color="category",
legend=dict(loc="center left", bbox_to_anchor=(1.0, 0.5)),
)
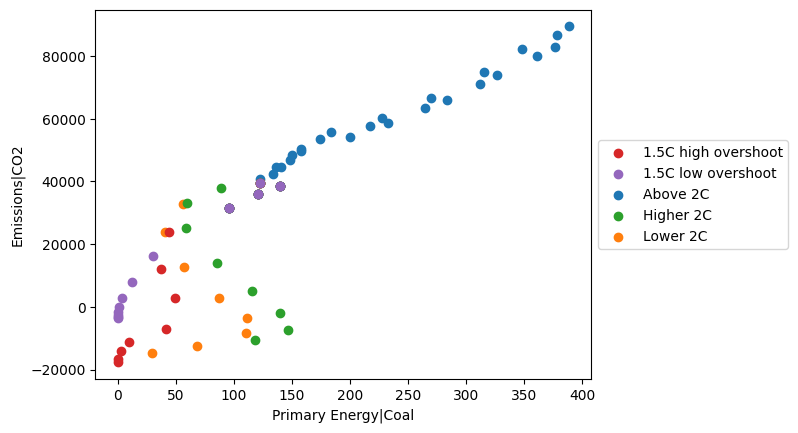
Exploring the data resource¶
If you’re interested in what data is available in the data source, you can use pyam.iiasa.Connection to do so.
[7]:
conn = pyam.iiasa.Connection("iamc15")
[INFO] 13:05:33 - pyam.iiasa: You are connected to the IXSE_SR15 scenario explorer hosted by IIASA. If you use this data in any published format, please cite the data as provided in the explorer guidelines: https://data.ece.iiasa.ac.at/iamc-1.5c-explorer/#/about
[INFO] 13:05:33 - pyam.iiasa: You are connected as an anonymous user
The Connection object has a number of useful functions for listing what’s available in the data resource. These functions follow the conventions of the IamDataFrame class (where possible).
A few of them are shown below.
[8]:
conn.models().head()
[8]:
0 AIM/CGE 2.0
1 AIM/CGE 2.1
2 C-ROADS-5.005
3 GCAM 4.2
4 GENeSYS-MOD 1.0
Name: model, dtype: object
[9]:
conn.scenarios().head()
[9]:
0 ADVANCE_2020_1.5C-2100
1 ADVANCE_2020_Med2C
2 ADVANCE_2020_WB2C
3 ADVANCE_2030_Med2C
4 ADVANCE_2030_Price1.5C
Name: scenario, dtype: object
[10]:
conn.variables().head()
[10]:
0 AR5 climate diagnostics|Concentration|CO2|FAIR...
1 AR5 climate diagnostics|Concentration|CO2|MAGI...
2 AR5 climate diagnostics|Forcing|Aerosol|Direct...
3 AR5 climate diagnostics|Forcing|Aerosol|MAGICC...
4 AR5 climate diagnostics|Forcing|Aerosol|Total|...
Name: variable, dtype: object
[11]:
conn.regions().head()
[11]:
0 World
1 R5ROWO
2 R5ASIA
3 R5LAM
4 R5MAF
Name: region, dtype: object
We queried the meta-indicator “category” in the above example, but there are many more. You can get a list with the following command:
[12]:
conn.meta_columns.head()
[12]:
0 Kyoto-GHG|2010 (SAR)
1 baseline
2 carbon price|2030
3 carbon price|2030 (NPV)
4 carbon price|2050
Name: name, dtype: object
You can directly query the Connection, which will return a pyam.IamDataFrame…
[13]:
df = conn.query(
model="MESSAGEix*",
variable=["Emissions|CO2", "Primary Energy|Coal"],
region="World",
)
…so that you can directly continue with your analysis and visualization workflow using pyam!
[14]:
ax = df.filter(variable="Primary Energy|Coal").plot(
color="scenario", legend=dict(loc="center left", bbox_to_anchor=(1.0, 0.5))
)
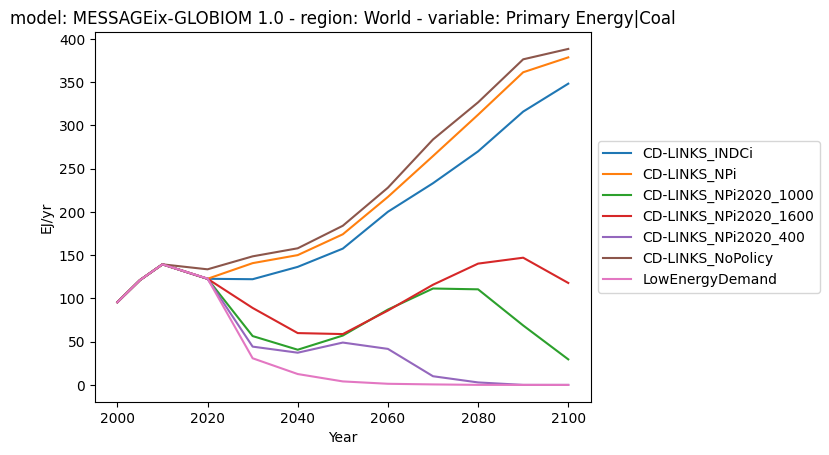
Loading all of a large database may take a few minutes on some connections. To save time when writing code you may reuse, you can save a local version of the database via the lazy_read_iiasa function. This is given a file location as well as whatever connection options we saw above. The first time the code is run, the result is stored there, and the code will read it from there on subsequent attempts.
[15]:
lazy_df = pyam.lazy_read_iiasa(
file="./tmp/messageix_co2_coal_data.csv",
name="iamc15",
model="MESSAGEix*",
variable=["Emissions|CO2", "Primary Energy|Coal"],
region="World",
)
[INFO] 13:05:42 - pyam.iiasa: You are connected to the IXSE_SR15 scenario explorer hosted by IIASA. If you use this data in any published format, please cite the data as provided in the explorer guidelines: https://data.ece.iiasa.ac.at/iamc-1.5c-explorer/#/about
[INFO] 13:05:42 - pyam.iiasa: You are connected as an anonymous user
[ ]: